Best PHP Code Review Practices
Let's be honest, code reviews can sometimes feel a bit tedious. But they're absolutely essential for creating PHP applications that work well, are easy to work with, and don't cause security headaches. The good news? There's a method to making code reviews effective. Let’s break down the key things to look for when reviewing PHP code.
Core Principles - 5 Pillars of Good PHP Code Reviews
Think of a code review as the quality assurance process for your PHP project. To safeguard the integrity and longevity of your application, we need a multi-faceted approach. Let's focus on these five essential pillars.
1. Does It Do the Job? Code Functionality Check
The most important aspect of a code review is making sure the code fulfils its intended purpose. Let's focus on the code's logic. Trace the flow of execution from the moment it receives input to the output it produces. Look for any illogical steps, incorrect calculations, or places where the process might unexpectedly stop.
- Check the inputs: Does the code properly handle all types of data it might receive? This includes user input, data from databases, or information from external systems.
- Inspect the outputs: Verify that the results produced by the code are both correct and in the expected format. Does the output data match the requirements?
Thorough testing is key to ensuring functionality. Unit tests help us systematically check individual components of the code with different input variations, ensuring the code behaves as expected in all scenarios.
During this step, I find it helpful to be able to release the code to a review app or staging server, and confirm my findings in code review with how it actually works. For tricky parts, I also tend to search for added Unit tests. If they are missing, it's probably a good idea to ask the author to add them.
2. Does It Work as Designed? Focusing on Code Functionality
At the core of a solid code review, we need to answer one fundamental question: does this code do what it's supposed to do? Begin by comparing the code directly with the project's requirements or specifications. Have you implemented all the necessary features? Are there incorrect behaviours or anything missing? Next, step through the code's logic carefully. Does the execution follow a sensible path from the input received to the final output? Look for any nonsensical branching (like if statements that are always false), infinite loops, or potential crashes.
Inspect how the code handles all forms of input. Will it work with different user entries, varied data pulled from a database, or information coming from another system? And just as importantly, is the output correct, formatted properly, and aligned with what the rest of your application anticipates?
Technical Tip: Don't just test by clicking around the application. While developers bear the primary responsibility for writing unit tests, don't underestimate the value of a critical eye during code review. As a reviewer, look for:
- Missing Tests: Are there blocks of code without corresponding unit tests?
- Edge Cases: Do the tests cover only expected scenarios, or do they include unexpected inputs and boundary conditions?
- Test Quality: Are the tests well-written and do they clearly assert the expected outcomes?
When reviewing, imagine ways a user could deliberately (or accidentally) try to break the code. Can you feed it strange inputs, cause unusual sequences of events, or overload it? Resilient code should handle these scenarios gracefully. Become proficient with a debugging tool like Xdebug. It lets you pause code execution, step through it line-by-line, and closely examine the values of variables as things change. For frontend code, I like to consider different UI states that might occur. Some key ones include empty state, loading state, and error state, but it's important to go further:
- Partially Loaded State: How is data displayed as it progressively loads? Are there clear loading indicators for different sections of the UI?
- Input Validation States: How does the UI immediately communicate success or failure of form validation (e.g., inline error messages)?
- Success States: After an action (e.g., submitting a form), how is success conveyed?
- Interaction States: Do elements provide visual feedback on hover, focus, or active states?
3. Can You Understand It? Prioritizing Code Readability
Readable code is essential for maintainability and collaboration. Let's focus on making your code easy for both humans and machines to parse. Start with strict adherence to coding standards like PSR-1 and PSR-12. These standards establish a common language for PHP code, defining rules for indentation, naming conventions, file organization, and more. By following standards, your code becomes predictable and consistent, reducing cognitive load for those reading it. Community standards like PSR minimize the learning curve for new developers joining a project and increase compatibility with different development tools.
During a code review, carefully assess variable and function naming. Do the names clearly convey their purpose, avoiding single-letter variables, unnecessary abbreviations, or vague terms? Well-named elements contribute to self-documenting code, minimizing the need for explanatory comments. If comments are present, do they focus on explaining the 'why' behind logic or design choices, rather than simply repeating what the code does?
If the code feels convoluted, suggest refactoring to the author. This could involve extracting methods, using more descriptive variable names, or restructuring code blocks for clarity. Emphasize the importance of long-term maintainability, even if it requires some additional effort in the present.
Utilize linters (like PHPCS) and static analysis tools (like PHPStan) as part of the review process. These tools help enforce standards, catch potential problems, and promote consistent readability. Look for mismatches between the code and established standards as potential areas for improvement.
If you find yourself struggling to understand a code flow during review, it's a strong indication that future maintainability will be a challenge. Don't hesitate to raise this with the author – collaborative discussion can often uncover better solutions or clarify the underlying logic.
In addition to formatting and naming, adhere strictly to project or company-specific coding rules. These cover aspects like namespaces, code organization, and architectural patterns. While automated tools can catch many violations, stay vigilant during your review for potential issues that tools might miss. This ensures consistency across the entire codebase.
4. Locking It Down: PHP Security Essentials
Web applications are prime targets for attacks. In the world of PHP, a secure code review would place special attention on a few critical areas. First and foremost, never trust data from external sources. Treat all user input (form submissions, URL parameters, etc.) as potentially malicious. Use PHP's built-in filter functions (filtervar, filterinput) to strip out dangerous characters (e.g., <script> tags to prevent XSS) and enforce rules to ensure the input matches what you expect (e.g., correct email format or valid numeric ranges). To protect your application from the notorious SQL injection vulnerability, avoid directly concatenating user input into SQL queries. Instead, rely on mysqli or PDO prepared statements (or even better, on database abstraction layers [DBAL] or some good ORM). They clearly separate the SQL structure from user-supplied data, allowing the database to handle the data safely and neutralize SQL injection attempts.
Finally, handle errors with care. Avoid displaying raw error messages (database errors, stack traces) to the user, as they could leak sensitive system information. Instead, log errors to a file for troubleshooting by developers, ensuring these logs are themselves protected from unauthorized access. When things go wrong, display generic, helpful error messages to the user, and log the details for internal debugging. In our case, we mostly use Monolog and forward logs to tools like DataDog or NewRelic. We also always have a Sentry instance connected to gather even more information on the issues.
While modern frameworks offer built-in security features, it's crucial to ensure their correct implementation during code review. Pay close attention to these aspects:
- Input Sanitization: Does the code meticulously filter and validate any data coming from users (forms, URL parameters, etc.)? Look for framework-specific input sanitization functions or methods.
- Prepared Statements: Are database queries consistently built using prepared statements? Check for framework methods that facilitate this protection against SQL injection.
- Error Handling: Does the code avoid exposing raw error messages or stack traces to the user? Are errors logged internally for developer troubleshooting? Is there a user-friendly fallback mechanism in case of failures?
Understanding these concepts empowers you to verify that the framework's safeguards are correctly in place, strengthening the application's overall security posture.
5. PHP Performance Optimization
Code that executes slowly frustrates users and can drain server resources. A thorough code review should always consider performance optimization, particularly focusing on these aspects:
- Smarter Algorithms: The way you structure your code has a huge impact on speed. Analyze your core algorithms and look for opportunities to use more efficient data structures (e.g., consider a hash table instead of nested loops for searching). Familiarity with Big O notation helps to understand how your code's efficiency scales with larger datasets.
- Database Interactions: Every query to the database adds overhead. Reduce unnecessary database calls by employing caching techniques (Memcached, Redis) to store frequently accessed data in memory. When you do need to query, optimize your SQL: use indexes appropriately, avoid fetching more data than you need, and be mindful of complex joins that might slow things down. Focus on indexes and query optimizations first, before you jump to using cache.
- Finding the Bottlenecks: Don't optimize blindly! Use profiling tools like Blackfire to get precise measurements of where your application spends most of its time. This pinpoints the functions or database queries that need the most attention. Blackfire provides valuable insights into execution time, function calls, and memory usage.
Technical Notes:
- Premature optimization is a trap: Focus on clean, functional code first. Over-optimizing too early can make code harder to read.
- Caching comes in many forms: Choose between in-memory caches, file-based caching, or even HTTP caching based on your application's needs.
- The larger the dataset, the bigger the algorithmic impact: Code that works fine with small-scale data might crawl as input sizes grow.
- Pay extra attention to database migrations. Pay close attention to database migrations, considering both code performance and the migration process itself. Large migrations can take considerable time (potentially even minutes), so understanding the potential impact upfront is crucial.
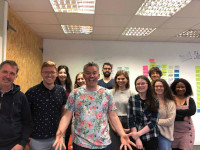
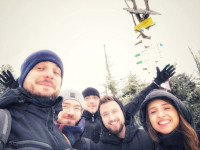
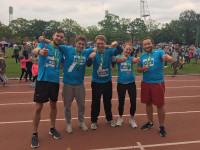
Accesto is a boutique software house focused on software craftsmanship and partnership. Founded in 2010 we have helped over 50 projects to overcome their technical challenges and outpace competition.
LEARN MOREBonus: Essential Checks for PHP Code Reviews
These additional areas deserve dedicated attention during your PHP code review process, even if they may not be as in-depth as the primary focus areas.
Dependency Management (Code Review Considerations)
While a thorough dependency audit is beyond the typical code review scope, here are key things to watch for:
- Critically Outdated Packages: Notice any major version discrepancies between installed packages and their latest releases. This might signal potential compatibility issues or security risks.
- Vulnerability Alerts: If you use tools like Snyk or Dependabot, check if they've flagged any known vulnerabilities in the project's dependencies.
- Versioning Implications: When suggesting package updates, be mindful of semantic versioning (Major.Minor.Patch) as major updates may have breaking changes.
- Company Standards: Some organizations have specific policies around dependency updates that reviewers should be familiar with.
- Review Scope: If time allows or security is a critical concern, a brief dependency scan with vulnerability-checking tools could be a valuable addition to a code review.
Architectural Adherence
If your project follows a defined architecture (e.g., MVC, layered architecture, etc.), closely assess if the code adheres to its principles. Key areas to consider:
- Separation of Concerns: Are responsibilities clearly distributed among different components? For example, avoid overloading models with complex business logic – consider services or dedicated classes for such logic.
- View Layer Integrity: Ensure presentation logic is confined to the view layer. Direct database queries or calculations in views violate separation and create testing difficulties.
- Architectural Guidelines: Refer to any project-specific documentation or established patterns outlining your chosen architecture. Modern web development employs a variety of architectural patterns. If you're unsure about the project's intended structure, discuss this with the author to better understand their design choices.
Database Interactions: Optimization and Security
Always ensure that database queries consistently use prepared statements to mitigate SQL Injection risks. Employ profiling tools integrated with your database (e.g., MySQL's slow query log) or extensions like Blackfire/New Relic to identify the most impactful optimizations. Pay attention to indexing – ensure appropriate indexes exist on frequently queried columns, particularly for tables with multi-column search criteria.
Error Handling
Define custom exception classes that create a hierarchy of errors (e.g., DatabaseException, ValidationException). This approach enables granular error handling throughout your codebase. Use different log levels (debug, info, warning, error) strategically. Configure your logging tools appropriately to store or alert based on severity. Finally, think carefully about error messages displayed to the user. These messages should clearly guide the user towards a solution without revealing sensitive system specifics.
Debugging issues reported by users can be tricky due to limited information. That's why clear error messages and detailed logs are essential. They provide the clues you need to quickly pinpoint problems and improve the user experience.
The Continuous Improvement Mindset: Code Reviews as a Habit
Code reviews shouldn't be seen as a one-time chore or a way to simply find bugs. By making them a regular practice within your development process, you'll consistently improve the quality of your PHP codebase. Each review session builds technical knowledge and strengthens collaboration within your team.
Code reviews are also a great way to transfer knowledge within a team. Not only will the developer coding a task know how it is implemented, but the person doing code review will also have a good understanding of it. In our case, we make sure each line added, removed, or changed is reviewed by at least one other person.
Remember, clean, secure, and well-structured code isn't just about aesthetics. It saves time debugging, reduces the risk of vulnerabilities, and allows your application to scale more gracefully. Make code reviews a non-negotiable part of your workflow. The benefits will compound over time, leading to more robust, maintainable, and successful PHP projects.