Is TypeScript Frontend or Backend?
TypeScript is a powerful language that's often mistaken for a frontend-only tool. But TypeScript is more than that. It's a superset of JavaScript, which means it can run on any JavaScript runtime, including Node.js. TypeScript also has a static type system, which can help you write more reliable code.
It's time that we explore the capabilities of TypeScript, examining its strengths and limitations in both frontend and backend domains. We'll also compare it to PHP and discover how TypeScript can empower PHP developers to seamlessly transition legacy code into the modern JavaScript ecosystem.
TypeScript as a Superset of JavaScript
Whilst not a programming language, TypeScript, a superset of JavaScript, seamlessly integrates with existing JavaScript code, allowing developers to leverage their existing JavaScript knowledge while enjoying the benefits of TypeScript's static type system. This compatibility ensures a smooth transition for developers familiar with JavaScript, as they can gradually incorporate TypeScript's type annotations into their codebase.
To illustrate the compatibility between TypeScript and JavaScript, consider the following example:
// JavaScript code
function greet(name) {
return "Hello, " + name + "!";
}
const greeting = greet("John Doe");
console.log(greeting); // Output: Hello, John Doe!
The above JavaScript code can be directly compiled and executed in TypeScript without any modifications. TypeScript recognizes the type annotations provided for the greet function's parameters and return value, enforcing type safety during development.
// TypeScript code
function greet(name: string): string {
return "Hello, " + name + "!";
}
const greeting: string = greet("John Doe");
console.log(greeting); // Output: Hello, John Doe!
The TypeScript code introduces a type annotation for the greeting variable, ensuring that it can only hold values of type string. This type constraint helps prevent errors like assigning a number value to greeting.
The benefits of TypeScript's static type system extend beyond error prevention. Type annotations provide clear documentation within the code itself, enhancing code readability and comprehension. This self-documenting nature makes it easier for developers to understand the intended usage of variables and functions, reducing the cognitive load during code reviews and maintenance.
To demonstrate the improved readability with type annotations, consider the following example:
// JavaScript code
function calculateArea(width, height) {
return width * height;
}
const area = calculateArea(10, 5);
console.log(area); // Output: 50
In this JavaScript code, the types of the width and height parameters are not explicitly defined, making it less clear what values are expected.
// TypeScript code
function calculateArea(width: number, height: number): number {
return width * height;
}
const area: number = calculateArea(10, 5);
console.log(area); // Output: 50
The TypeScript code explicitly defines the types of the width and height parameters as number, and also specifies that the return value is of type number. This type information enhances the code's readability, making it easier for developers to understand the intended usage of the function.
TypeScript for Frontend Development and Frontend Developers
TypeScript has firmly established itself as a staple in modern frontend development, seamlessly integrating with popular JavaScript libraries and frameworks like Angular, React, and Vue.js and is often mistaken as being a programming language. Its static type system brings a new level of code reliability, maintainability, and error detection to frontend applications, enhancing the overall development experience.
TypeScript in Angular
Angular, a powerful and versatile frontend framework, embraces TypeScript as its primary language through its TypeScript support. TypeScript's type annotations play a crucial role in Angular's component-based architecture, ensuring type safety across components, services, and directives. This type-driven approach leads to fewer runtime errors, improved code readability, and enhanced maintainability of large Angular applications.
To illustrate the use of TypeScript in Angular, consider the following example of a component with type annotations:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-product-details',
templateUrl: './product-details.component.html',
styleUrls: ['./product-details.component.css']
})
export class ProductDetailsComponent implements OnInit {
product: Product; // Type annotation for 'product' property
constructor() { }
ngOnInit(): void {
// Fetch product data and assign it to 'product' property
}
}
In this example, the product property of the ProductDetailsComponent class is explicitly defined as having the type Product. This type annotation ensures that only values of type Product can be assigned to the product property, preventing errors related to incompatible data types.
TypeScript in React
React, another widely used frontend library, has also adopted TypeScript as a compatible language. TypeScript's type system complements React's component-based approach, providing type safety for props, state, and component lifecycle methods. This helps prevent errors related to incorrect prop types, state mutations, and lifecycle misconfigurations, leading to more robust and reliable React applications.
To demonstrate TypeScript's type annotations in React, consider the following example of a functional component:
import React from 'react';
interface ProductProps {
id: number;
name: string;
price: number;
}
function ProductDetails(props: ProductProps) {
const { id, name, price } = props; // Type annotations for destructured props
return (
<div>
<h2>Product Details</h2>
<p>ID: {id}</p>
<p>Name: {name}</p>
<p>Price: ${price}</p>
</div>
);
}
export default ProductDetails;
In this example, the ProductDetails function accepts a props object of type ProductProps. The ProductProps interface defines the expected properties and their types, ensuring that the component only receives props of the correct data types. This type-driven approach helps prevent errors related to incorrect prop usage and data type mismatches.
TypeScript in Vue.js
Vue.js, a progressive and lightweight frontend framework, also benefits from TypeScript's integration. TypeScript's type annotations enhance the Vue.js development process by providing type safety for data bindings, computed properties, and component lifecycle hooks. This type-aware approach helps prevent errors related to data type mismatches, invalid computations, and premature component destruction, leading to more reliable and maintainable Vue.js applications.
To illustrate TypeScript's type annotations in Vue.js, consider the following example of a component with computed properties:
<template>
<div>
<h2>Product Details</h2>
<p>ID: {{ product.id }}</p>
<p>Name: {{ product.name }}</p>
<p>Price: {{ product.price }}</p>
<p>Product Category: {{ productCategory }}</p>
</div>
</template>
<script>
export default {
computed: {
productCategory() {
// Computed property logic using product data
return 'Electronics'; // Type annotation for return value
}
}
};
</script>
In this example, the productCategory computed property is defined with a type annotation, ensuring that its returned value is of type string. This type annotation helps prevent errors related to incompatible data types and ensures that the computed property's value is always a string.
TypeScript for Backend Development
TypeScript's impact extends beyond frontend development, making its mark in the realm of backend development as well. TypeScript's static type system proves invaluable in building secure, scalable, and maintainable backend APIs, leveraging the power of JavaScript runtime environments like Node.js.
Benefits of TypeScript in Backend Development
Improved Type Safety: TypeScript's type annotations provide type safety to backend code, ensuring that variables, functions, and API endpoints handle data according to their defined types. This type-driven approach helps prevent runtime errors and unexpected behavior, leading to more robust and reliable backend applications.
Consider the following example of a TypeScript function that fetches user data from an API:
async function fetchUserData(userId: number): Promise<User> {
const response = await fetch(`https://api.example.com/users/${userId}`);
const userData = await response.json();
return userData as User; // Type annotation for returned value
}
In this example, the fetchUserData function explicitly defines the userId parameter as having the type number, ensuring that only valid user IDs are used to fetch data. The type annotation for the returned value, Promise
Enhanced Security: TypeScript's type system helps prevent malicious input and data injection attacks, making backend applications more secure. By enforcing type constraints, TypeScript ensures that data is handled according to its intended purpose, reducing the risk of security vulnerabilities.
Consider the following example of a TypeScript function that sanitizes and validates user input:
function sanitizeUserInput(input: string): string {
// Sanitize and validate input to prevent malicious code injection
return sanitizedInput; // Type annotation for returned value
}
In this example, the sanitizeUserInput function sanitizes and validates user input before returning it. By explicitly defining the input parameter as having the type string, TypeScript ensures that only valid string input is processed, reducing the risk of security vulnerabilities.
Scalable API Development: TypeScript facilitates the development of scalable and maintainable backend APIs by providing clear type definitions for API endpoints, request data, and response types. This type-aware approach promotes better understanding of API interactions and reduces the risk of errors when integrating with external services.
Consider the following example of a TypeScript API endpoint that creates a new user:
app.post('/users', async (req, res) => {
const newUser: User = {
name: req.body.name,
email: req.body.email,
// ... other user data fields
};
// Validate and process new user data
// Send response with appropriate status code and message
});
In this example, the API endpoint explicitly defines the newUser object as having the type User, ensuring that only valid user data is extracted from the request body. This type-safe approach helps prevent errors related to invalid data types and promotes better understanding of the API's data expectations.
Comparative Analysis: TypeScript vs. PHP
TypeScript and PHP are both programming languages widely used in web development, each with its own unique strengths and limitations. TypeScript, a superset of JavaScript, embraces a static type system, while PHP employs dynamic typing. This fundamental difference in their type systems leads to distinct advantages and challenges for each language.
Static Typing vs. Dynamic Typing
JavaScript is dynamically typed by default. TypeScript's static type system enforces type constraints during development, ensuring that variables, functions, and expressions adhere to their defined types. This type-driven approach promotes code reliability, maintainability, and error detection, making TypeScript well-suited for complex web applications with stringent requirements.
PHP, on the other hand, employs dynamic typing, where type checking is performed at runtime. This approach offers flexibility and ease of development, but it can also lead to runtime errors and unexpected behavior if type mismatches occur.
Frontend Development
In frontend development, TypeScript's static type system proves valuable in building large, complex Single-Page Applications (SPAs). Its ability to prevent type-related errors early on reduces debugging time and improves code maintainability. Popular frontend frameworks like Angular, React, and Vue.js integrate seamlessly with TypeScript, leveraging its type system to enhance code reliability.
PHP, while not as commonly used for frontend development, can still be employed for simpler frontend tasks or in conjunction with server-side rendering frameworks like Laravel Blade. However, the lack of static type checking can make it more challenging to maintain large frontend codebases in PHP.
Backend Development
In backend development, TypeScript's type system plays a crucial role in building secure and scalable APIs for backend developers. Whilst not traditionally considered a backend language, by enforcing type constraints, TypeScript helps prevent malicious input and data injection attacks, making backend applications more secure. Additionally, TypeScript's type annotations provide clear documentation within the code itself, enhancing code readability and maintainability.
PHP, with its dynamic typing approach, offers flexibility and rapid development for backend applications. However, the lack of static type checking can lead to runtime errors and security vulnerabilities if not carefully managed.
TypeScript and PHP: Bridging the Gap
TypeScript and PHP, two prominent languages in the web development realm, may seem like distant cousins. TypeScript, a superset of vanilla JavaScript, embraces static typing, while PHP employs dynamic typing. Yet, despite their differences, these languages can coexist and interoperate, offering a powerful combination for web, frontend and backend development.
Leveraging TypeScript for PHP APIs
In our approach, TypeScript significantly enhances the development of PHP APIs by introducing a layer of type safety and improved code maintainability through the use of DTO (Data Transfer Object) patterns. This method involves defining DTO objects like UserDTO as contracts between frontend and backend developers. By agreeing on these DTO objects, TypeScript ensures type compatibility and streamlines the integration process between the frontend TypeScript code and backend PHP services.
Consider the following example of a TypeScript API endpoint written to interact with a PHP backend:
import axios from 'axios';
interface UserDTO {
id: number;
name: string;
email: string;
}
async function fetchUserData(userId: number): Promise<UserDTO> {
const response = await axios.get(`https://api.php.example.com/users/${userId}`);
const userData = response.data;
return userData as UserDTO; // Type annotation for returned value
}
In this example, the fetchUserData function explicitly defines the userId parameter as having the type number, ensuring that only valid user IDs are used to fetch data. The type annotation for the returned value, Promise
Integrating TypeScript with PHP Frameworks
TypeScript can be seamlessly integrated with popular PHP frameworks, especially Symfony, which is our main framework. Using TypeScript with Symfony, along with Twig templates for server-side rendering, ensures a robust development process. This integration allows developers to enforce type compatibility between Symfony objects and TypeScript code, greatly improving code maintainability and reducing the risk of type mismatches.
For instance, consider the following example of a TypeScript function interacting with a Symfony model:
import { UserDTO } from './UserDTO';
async function getUserById(id: number): Promise<UserDTO> {
const response = await fetch(`https://api.symfony.example.com/user/${id}`);
const symfonyUser = await response.json();
const mappedUser: UserDTO = {
id: symfonyUser.id,
name: symfonyUser.name,
email: symfonyUser.email,
};
return mappedUser;
}
In this example, the getUserById function explicitly defines the id parameter as having the type number, ensuring that only valid user IDs are used to fetch data. The type annotation for the returned value, Promise
While TypeScript and PHP may have different type systems, they can effectively collaborate in web development projects. By leveraging TypeScript's type annotations for PHP APIs and integrating TypeScript with PHP frameworks, developers can benefit from the strengths of both languages, enhancing code reliability, maintainability, and security. This hybrid approach opens up new possibilities for web development, combining the flexibility of PHP with the type safety of TypeScript, creating a powerful synergy for building robust and maintainable web applications.
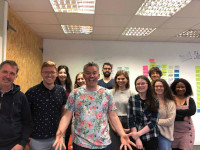
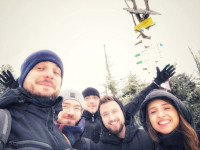
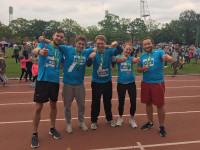
Accesto is a boutique software house focused on software craftsmanship and partnership. Founded in 2010 we have helped over 50 projects to overcome their technical challenges and outpace competition.
LEARN MORESometimes, two is better than one
TypeScript and PHP, two languages that might seem different, can work together well to create modern web applications for the frontend and backend. TypeScript's type system helps catch errors early and makes code easier to maintain, while PHP is fast and flexible.
Together, TypeScript and PHP can make web development projects more reliable and easier to manage. This combination can help developers build websites and apps that are both powerful and easy to use.
So, if you're a developer looking for a new way to build web applications, consider using TypeScript and PHP together. You might be surprised at how well they work together.